Chatbots are viewed as self-serving tools helping users to get answers to their inquiries.
Today, these bots are utilized for a variety of purposes. Apart from obvious cases - sales and support inquiries, sometimes chatbots are used by companies’ internal issues and solve organizational issues.
In our case, we use a time-tracking chatbot which allows monitoring the development workflow and evaluating each company member performance. Today we’re going to show you how we created this bot (we call it ‘Timebot’) and what benefits it brings to our company’s workflow.
The challenge
Time tracking is a part of everyday life in any software development project, for both internal and external purposes. For example, we need to send reports to our clients, to monitor the team workload and redistribute the tasks accordingly.
With the company’s growth, the scope of our projects became more extensive, and all of them became pretty diverse. Thus, monitoring the development workflow and evaluating each company member performance became an important issue for us. So we started looking for options to automate reporting and simplify the time tracking process.
At Codica, we utilize Slack for corporate communication to discuss all project issues. And the Slack App provides an opportunity to create different bots which help to automate everyday routine. Naturally, we had an idea to create a Slack bot for time tracking.
Additionally, one of the leading grounds to build a time-tracking tool using Slack is that web solutions are rather time-consuming. We were interested in creating a more effective and optimized way of time-tracking.
When we made the decision to create our Slack bot for time tracking, we proceeded to gather requirements. The solution was not supposed to work for time tracking only, so we had to define all the issues it would help us solve.
The solution
Requirements
At the beginning of the bot development, we were looking to cover the following needs:
- Simplify the tracking process for the team
- Automate reporting system for customers
- Provide the opportunity to clearly evaluate the overall workload
- Record each company’s employee contribution to the projects
After initial discussions, we arrived at the idea that the following features list would help us with the above requirements:
Time tracking would allow tracking the number of hours spent on a particular task or project.
Transparent reporting system would allow gathering and getting particular information for a specific period from the timesheets and provide the customers with timely and accurate reports.
Bundling with Trello would simplify the process of time tracking. We have chosen the Trello system because we utilize it for internal task management and tracking. We would be able to insert a particular Trello ticket for time tracking or messaging.
Administration panel would give an opportunity to monitor the company employees’ timesheets, current projects they work on, absence days etc. Also, it would simplify tracking the overall performance.
Development
1. Defining the technology stack
After considering the required functionality, we turned to technical implementation. For development purposes, we have created two applications:
As for the technology stack, we have chosen the following tools for Slack bot:
- Rails 5
- Ruby 2.4
- PostgreSQL
And these technologies were used for Admin panel development:
- Vue
- Vue-router
- Vuex
- Vue-element-admin (admin panel template)
- JWT (JSON Web Token)
The main reason to use such technologies as Vue.js and Rails is that we like them and utilize in our development process.
2. Bot creation and integration
Initially, we have created a bot at Slack API following the setup instructions. You set your workspace, define Bot’s name, profile picture and get a special API token.
For the purposes of API management for our chatbot, we have utilized Slack Ruby Client to use the special interface.
Thus, we have added the Slack API token to the .env
file (create it if you don’t have one) the following way:
Slack.configure do|config|
config.token =ENV['SLACK_API_TOKEN']
End
This way we have integrated Slack API and now we can configure the bot.
Let’s connect and discuss what features your chatbot should have.
3. Creating the list of commands
When the Slack Bot was ready for configuration, we have turned to create the available commands. All of them were created through if..else
conditional statements. Here are some commands from the list:
Commands | Description |
help | print help. |
projects | print all available projects. |
find project SEARCH_QUERY | find specific project. |
PROJECT_NAME HOURS:MINUTES COMMENT | log time. |
/logtime | log time via interective dialogue. |
edit NEW_DATE(OPTIONAL) TIME_ENTRY_ID HOURS:MINUTES COMMENT | edit an existing time entry. |
update(OPTIONAL) DAY.MONTH.YEAR PROJECT_NAME HOURS:MINUTES COMMENT | create an entry for the specific date. |
add project PROJECT_NAME | add new project. |
show day/show week/show month | get a report for this day/week/month. |
show absence/show absence last year | display absences. |
set REASON DAY.MONTH.YEAR(OPTIONAL) - DAY.MONTH.YEAR(OPTIONAL) COMMENT(OPTIONAL) | if you were/are absent on some days. |
Each user is assigned a special ID to be defined by the bot. When a user enters a command, the bot checks whether it’s a valid command. If ‘true’, the bot returns the following data for this particular user by making a request to the database. If ‘false’, the bot returns ‘I don’t understand you’.
First of all, we’ve created the Event Handler:
classEventHandlerinclude Message::Conditions
include Message::Logger
attr_reader :client,:data,:sender,:messages,:public_channelsdefinitialize(client, data, messages, public_channels)@client= client
@data= data
@public_channels= public_channels
@sender= Message::Sender.new@messages= messages
enddefhandle_messagereturnif message_is_not_processable
user = User.find_by(uid: data.user)
log_incoming_message(user, data.text)if message_is_enter_time
CreateEntry.call(user, data.text, messages)elsif message_is_specify_project
SpecifyProject.call(user, data.text, messages)elsif message_is_request_for_help
ShowHelp.call(user)elsif message_is_remove_entry
RemoveEntry.call(user, data.text)elsif message_is_show_reports
ShowReport.call(user, data.text)elsif message_is_enter_time_for_day
CreateEntryForDay.call(user, data.text)elsif message_is_request_for_project
ShowProjects.call(user)...else
DoNotUnderstand.call(user, messages)endend
End
Then, we’ve created Classes for every bot’s command, for example:
classAddProject< BaseService
include ServiceHelper
attr_reader :user,:textdefinitialize(user, text)@user= user
@text= text
super()enddefcall
project_name = text.match(Message::Conditions::ADD_PROJECT_REGEXP)[1]
project = find_project_by_name(project_name)if project
sender.send_message(user,"Project with name #{project.name} already exists.")returnendif project_name.length < Project::MINIMUM_PROJECT_NAME_LENGTH
text ="Project name is too short - must be at least #{Project::MINIMUM_PROJECT_NAME_LENGTH} characters."
sender.send_message(user, text)returnend
project = Project.create!(name: project_name)
sender.send_message(user,"Project with name #{project.name} is created.")endend
It’s possible to add new commands anytime.
Examples of our Timebot workflow
Time tracking allows tracking the number of hours spent on a particular project and monitoring a development workflow as a whole. The information will reflect on each member’s timesheet, which can be later viewed and edited.
To log time, you need to enter the project name, time spent for a particular task, and add comments to specify the task details.
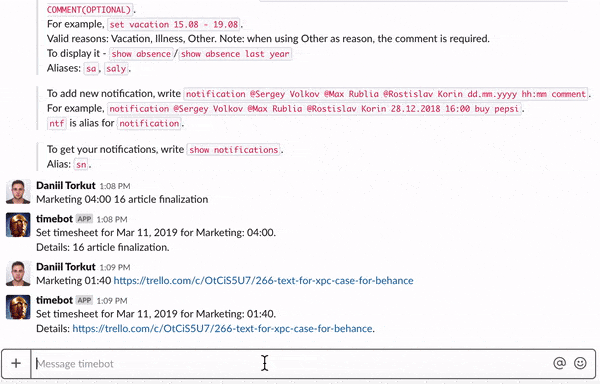
Absences allow tracking employee’s vacation, illnesses, and other reasons for absence on a particular day.
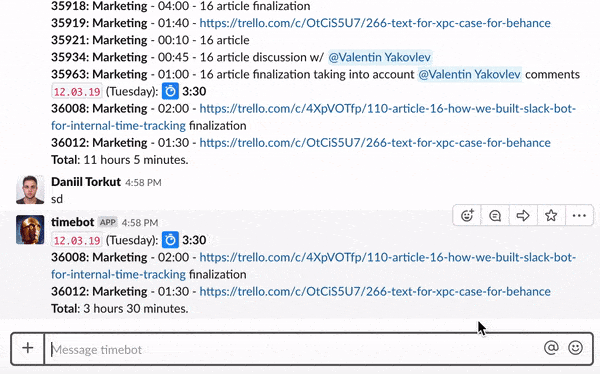
Reports are convenient to get particular data and gather it for further statistics creation and analysis.
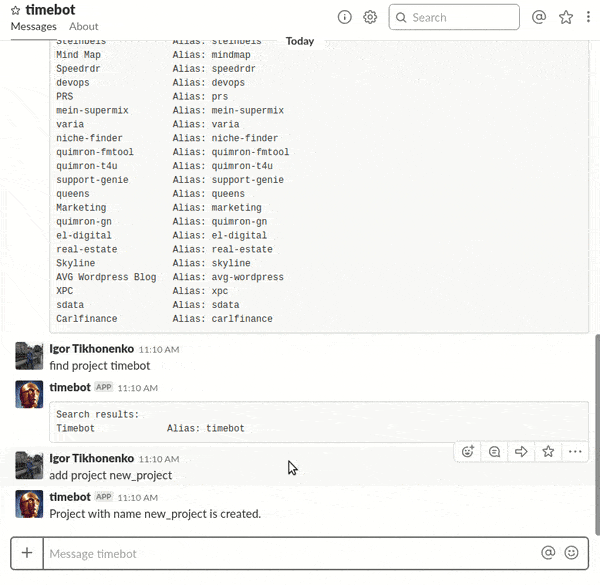
Projects are implemented to allow the users to monitor, add, and utilize the project for time logging.
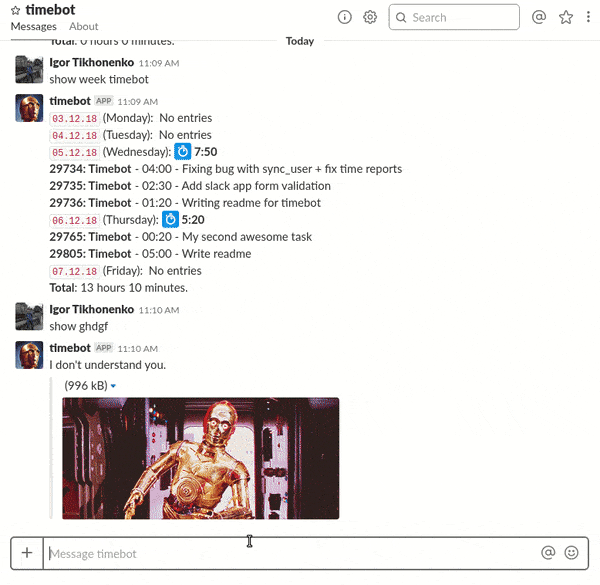
Administration panel
Along with the Slack bot, we have developed an Administration panel. It gives an opportunity to monitor the company members’ timesheets, current projects they work on, absence days, etc.
It is the internal part of our time tracking tool and is particularly valuable for project managers. It fully reflects the overall performance statistics on individuals, teams, and projects at any chosen period.
In the Dashboard, we have a visual display of our data. The graphs contain the number of hours spent by a user, position, for a specific project. Moreover, the Dashboard allows getting information about the projects particular users are working on.
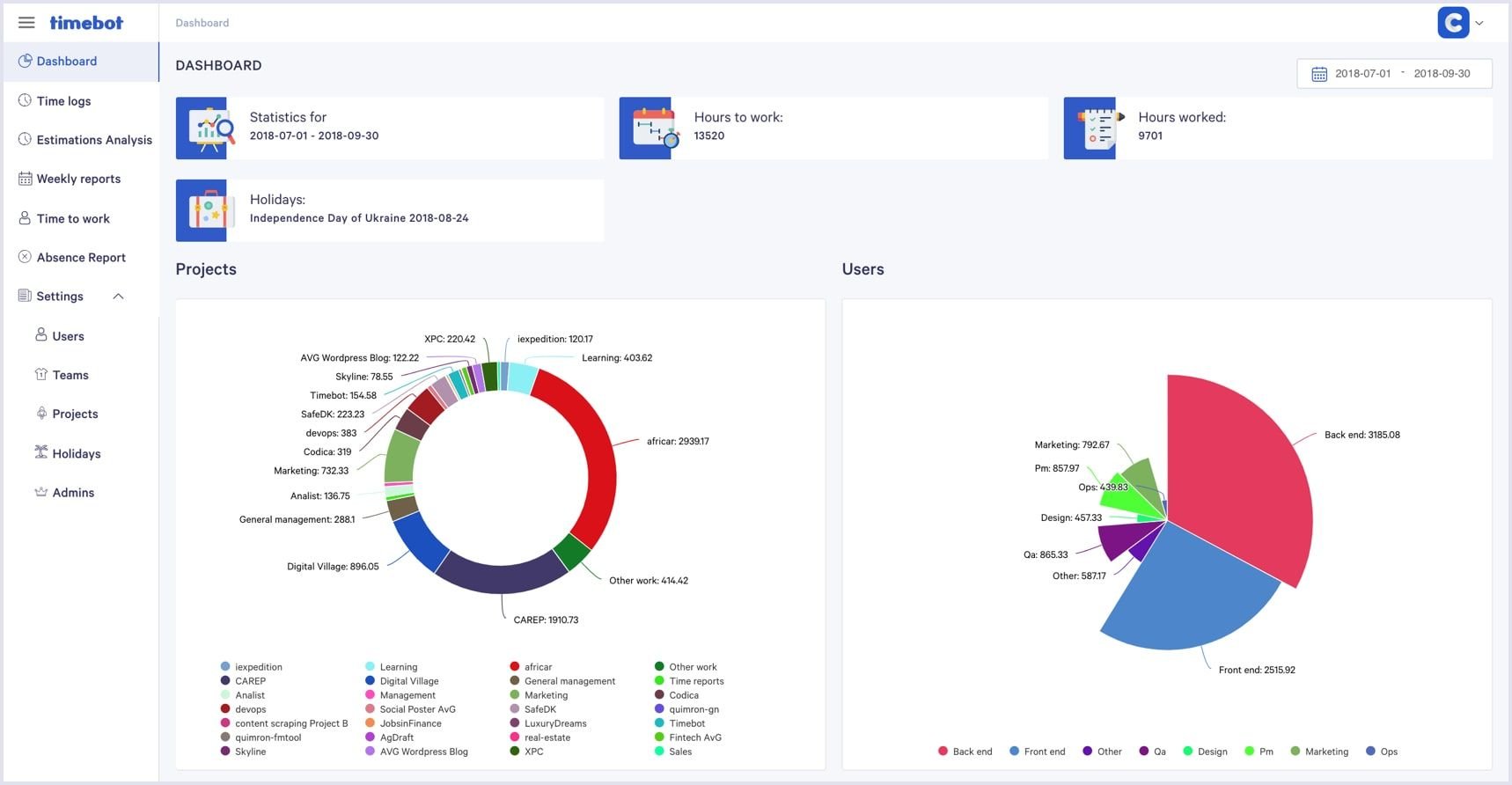
Also, here is our Time logs section which allows monitoring, viewing, editing, and deleting the data of logged time.
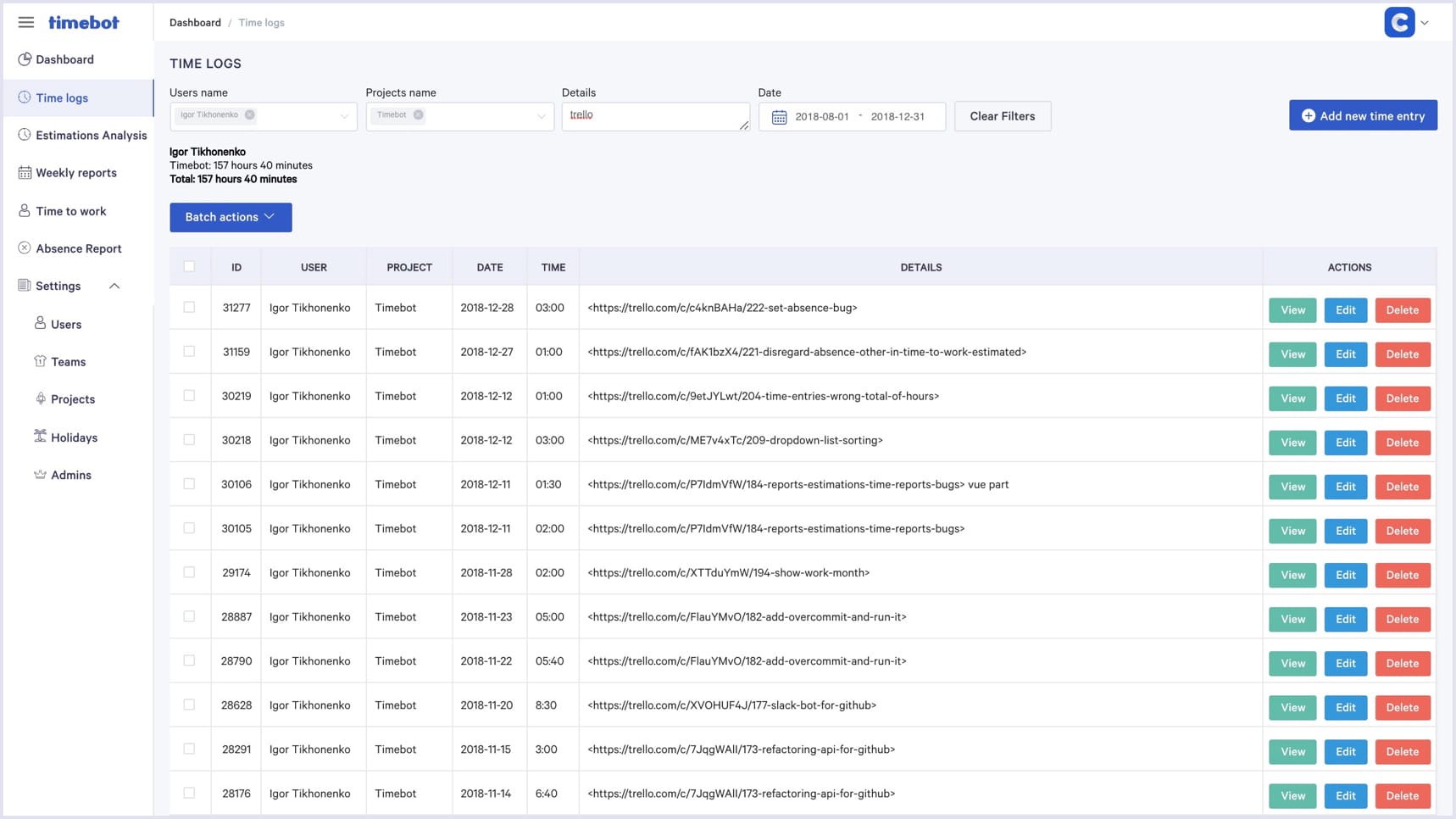
The results
We have developed a time-tracking tool in Slack, which fully meets our requirements and needs. The main benefits of Timebot creation are easier monitoring of the development workflow and evaluating each company member performance.
Here is the full list of benefits and features that our Timebot brings:
- Tracking of time worked on a specific project
- Tracking absences (vacation, sick leave)
- Notifications with meeting time that pops up in 10 minutes before the meeting, discussion
- Performance and velocity of the internal company processes
- Analysis of time tracked depending on the position like front-end and back-end developer, QA, PM that sufficiently helps project managers in estimating
- The accuracy of reports and statistics that we provide to the customers
To conclude, we have shown you how to create a SlackBot for time tracking, and hope that this guide will be interesting for you and your business. We would add that chatbots are handy and powerful tools for different tasks you may create.